Recurring Billing
Integrate the recurring billing APIs to automated your subscription payments
Recurring Billing APIs are the simplest way to start collecting your monthly or yearly subscription fees automatically.
This API integration includes 4 Steps
Create a Subscription Plan
The first step is to create a subscription plan with details and pricing.
Set Up Recurring Billing
Once the subscription plan is defined, set up recurring billing for the customer.
Present the Checkout UI
Present the checkout user interface to the customer for subscription confirmation.
Handle Payment Processing
After the customer confirms, handle payment processing and schedule recurring charges.
Step 1 - Create a subscription plan
A subscription plan consists of basic information like plan name, amount, and frequency. An example of a subscription plan could be “Spotify Premium” which is SGD 9.90 monthly.
HTTP Request
POST https://api.sandbox.hit-pay.com/v1/subscription-plan
Query Parameters
Mandatory fields are name
, cycle
and amount
. Remember to include header Content-Type: application/x-www-form-urlencoded
Parameter | Description | Example |
---|---|---|
name | Plan name | Spotify Premium |
description | The description of the subscription plan | Spotify Monthly Subscription |
cycle | Billing frequency (weekly / monthly / yearly / custom / save_card). If cycle = custom then the user has to send the fields cycle_repeat and cycle_frequency | monthly |
cycle_repeat | [This field is only applicable when cycle = custom] It’s the number of times the cycle will repeat. | 4 |
cycle_frequency | [This field is only applicable when cycle = custom] It’s the frequency of the cycle [day / week / month / year] | week |
currency | Currency related to the recurring billing | SGD |
amount | Amount related to the recurring billing | 9.90 |
reference | Arbitrary reference number that you can map to your internal reference number. This value cannot be edited by the customer | XXXX123 |
Response
{
"id": "973ee344-6737-4897-9929-edbc9d7bf433",
"name": "Spotify Premium",
"description": "Spotify Monthly Subscription",
"cycle": "monthly",
"cycle_repeat": null,
"cycle_frequency": null,
"currency": "sgd",
"amount": 9.90,
"reference": "spotify_premium_2022",
"created_at": "2022-09-12T14:19:26",
"updated_at": "2022-09-12T14:19:26"
}
Step 2 - Create a recurring billing for the customer
Once your customer has decided to start the subscription this endpoint will create the recurring billing request.
Since this is a server-to-server communication, if you have a mobile or Web client that communicates with your REST API, you must have a new endpoint E.g. /create-subscription or reuse an existing endpoint. This endpoint will be responsible for making the recurring billing API call to hitpay.
HTTP Request
POST https://api.sandbox.hit-pay.com/v1/recurring-billing
Query Parameters
Mandatory fields are plan_id
, customer_email
, and start_date
.
If you would like to create a subscription without a plan, you need to send plan_id = null
and the following mandatory fields: name
, cycle
, amount
, customer_email
, and start_date
. Remember to include the header Content-Type: application/x-www-form-urlencoded
.
Parameter | Description | Example |
---|---|---|
plan_id | Subscription plan id created from step 1 | 973ee344-6737-4897-9929-edbc9d7bf433 |
customer_email | Customer email | paul@hitpayapp.com |
customer_name | Customer name | Paul |
start_date | Billing start date (YYYY-MM-DD) in SGT | 2022-11-11 |
amount | By default, the amount from the subscription plan will be used. Use this parameter for discounts for customers. | 7.90 |
currency | Currency related to the recurring billing | SGD |
payment_methods[] | Choice of payment methods you want to offer the customer | giro, card |
redirect_url | URL where hitpay redirects the user after the user enters card details and the subscription is active. | https://spotify.com/subscription-completed |
reference | Arbitrary reference number for mapping purposes. Customer cannot edit this value. | XXXX123 |
webhook | Optional URL for hitpay to send a POST request on new charges or charging errors. | https://webhoo.site/test |
send_email | Hitpay sends email receipts to the customer. Default value is false. | true |
times_to_be_charged | Number of times to charge the customer. | 3 |
Step 3 - Redirect customer to recurring billing page (One time set up)
Redirect the customer to the “url” value.
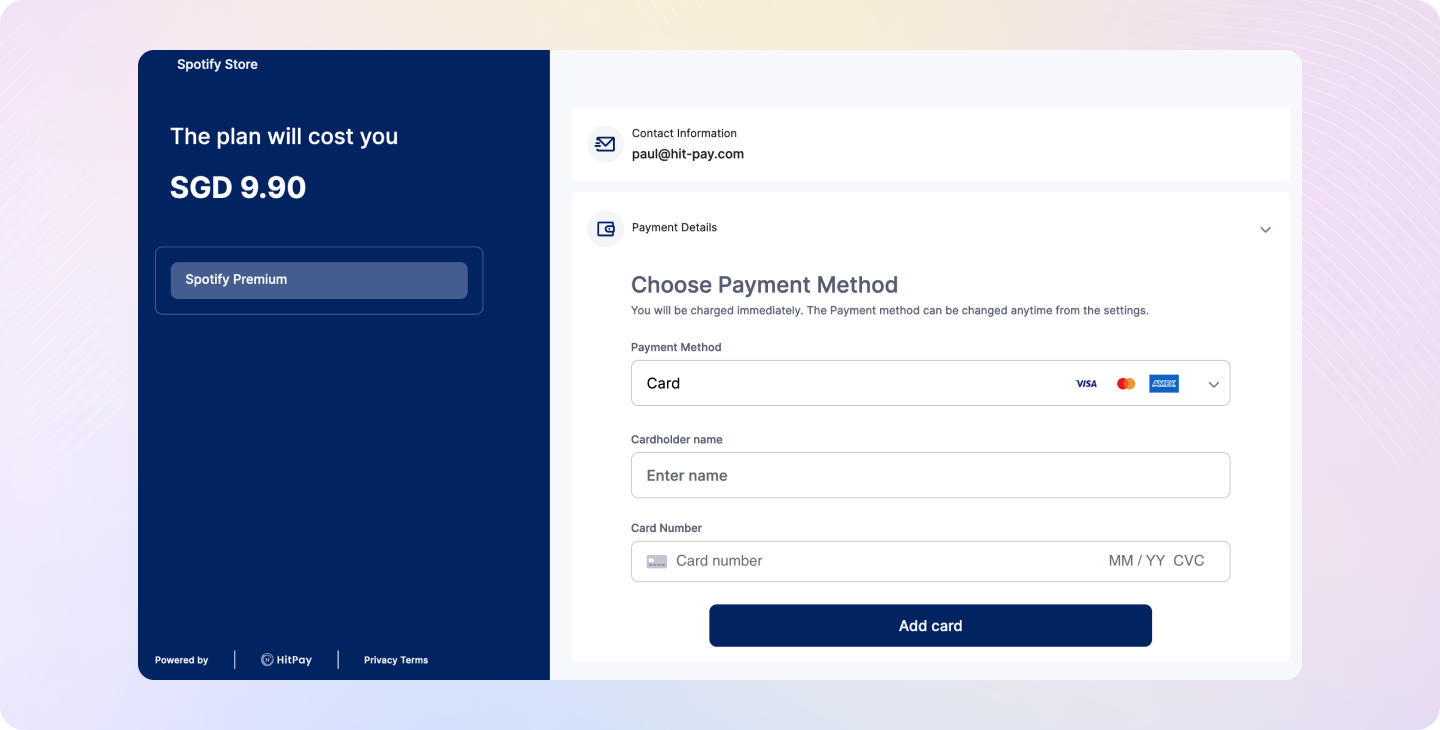
Once the customer completes the payment information it will be redirect to “redirect_url”
Step 4: Handle Successful Payment
Webhooks
HitPay will send a Webhook POST request when there is a new charge or if there is an error charging the card.
If you are using HitPay APIs to integrate into your app you must mark your order as paid ONLY after the webhook is received and validated.
- Create an endpoint (E.g. /payment-confirmation/webhook) in your server that accepts POST requests. This request is
application/x-www-form-urlencoded
. - Validate the webhook data using your salt value
- Return HTTP status code 200 to Hitpay
- Mark your order as paid
payment_id=974f65d6-88ea-42a0-a67a-15acafc4dc66&recurring_billing_id=9741164c-06a1-4dd7-a649-72cca8f9603a&amount=9.90¤cy=sgd&status=succeeded&reference=cust_id_123&hmac=7690ff7ab7d88480a480b8be722f6dd12cc58f489da25c504dbe29c04b297418
Webhook fields
Following fields are sent with the webhook request:
Parameter | Description |
---|---|
payment_id | Payment ID |
recurring_billing_id | Recurring billing request ID |
amount | Amount related to the recurring billing |
currency | Currency related to the recurring billing |
status | Payment status (succeeded / failed) |
reference | Arbitrary reference number that you have mapped during recurring billing request creation |
hmac | Message Authentication code of this webhook request |
Validate Webhook
Hitpay creates a list of all values from the key-value pairs that we send in the POST request and sort them in the order of their keys alphabetically. We then concatenate all these values together. We then use the HMAC-SHA256 algorithm to generate the signature. The HMAC key for the signature generation is the secret salt
from your dashboard under API Keys
.
public function generateSignatureArray($secret, array $args)
{
$hmacSource = [];
foreach ($args as $key => $val) {
$hmacSource[$key] = "{$key}{$val}";
}
ksort($hmacSource);
$sig = implode("", array_values($hmacSource));
$calculatedHmac = hash_hmac('sha256', $sig, $secret);
return $calculatedHmac;
}
Congrats! You have now successfully completed the recurring billing integration.
FAQs
Was this page helpful?